Mastering Angular Data Binding: A Comprehensive Guide for Developers
With data binding, you can effortlessly link data between your application's components and its views, making it a core aspect of Angular development.
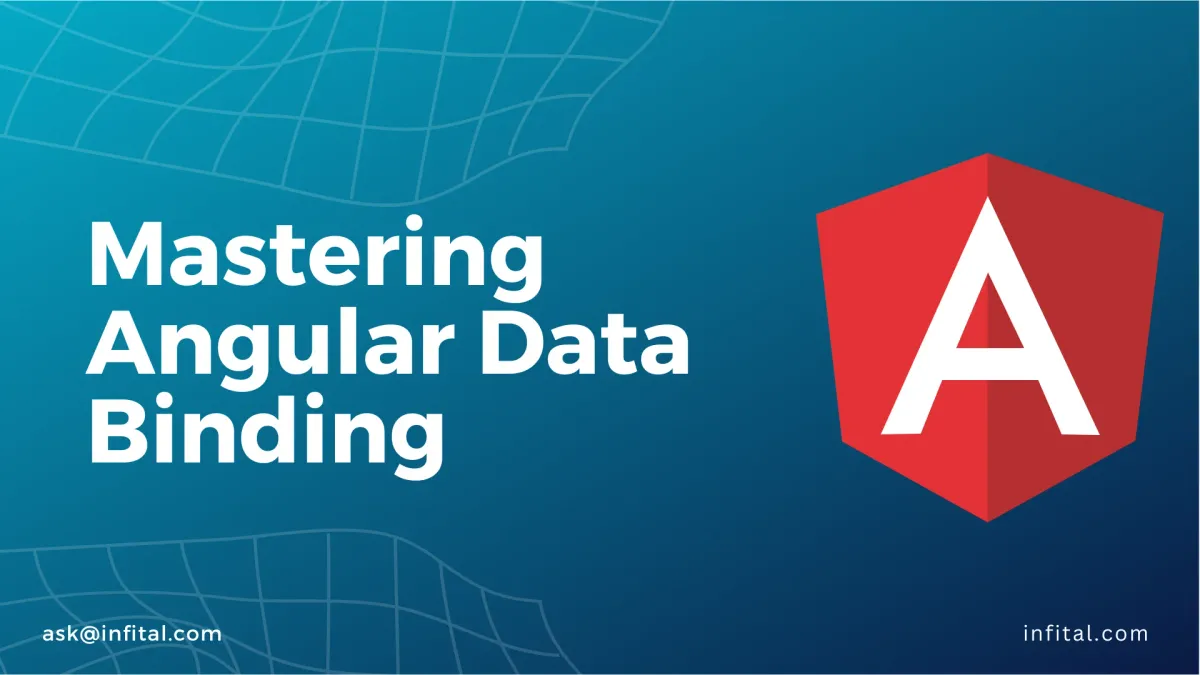
Angular data binding is a powerful feature that revolutionizes the way developers handle data in web applications. With data binding, you can effortlessly link data between your application's components and its views, making it a core aspect of Angular development. In this comprehensive guide, we will delve into the two primary data binding techniques - One-Way Data Binding and Two-Way Data Binding. We will explore the intricacies of each method, understand how they work, and provide practical coding examples to demonstrate their usage. Let's embark on this journey to harness the full potential of Angular data binding.
Understanding One-Way Data Binding
One-Way Data Binding is a fundamental concept in Angular, enabling the flow of data from a component to the HTML view. It means that data moves in a single direction, from the component to the view, ensuring a unidirectional flow of information. This technique is ideal for displaying static data in the view or when you want to update the UI based on changes in the component.
Example: Let's explore a basic example of one-way data binding. Imagine we have a component with a property called "message" that we want to display in the view.
app.component.ts:
export class AppComponent {
message = "Welcome to Infital!";
}
app.component.html:
<h1>{{ message }}</h1>
In this example, the "message" property is bound to the <h1>
tag using double curly braces {{ }}. As a result, the message "Welcome to Infital!" will be displayed on the web page.
Unveiling Two-Way Data Binding
Two-Way Data Binding is a more dynamic approach where data flow occurs both from the component to the view and from the view back to the component. It allows synchronization between the model and the view, ensuring any changes made by the user in the view are instantly reflected in the component and vice versa. This technique is commonly used in forms and interactive applications.
Example: To demonstrate two-way data binding, let's create a simple input field that updates the component property as the user types.
app.component.ts:
export class AppComponent {
name = "Sithira Senanayake";
}
app.component.html:
<input [(ngModel)]="name" placeholder="Enter your name">
<h2>Hello, {{ name }}!</h2>
In this example, we use the "ngModel" directive along with the banana-in-a-box syntax [( )] to achieve two-way data binding. The "name" property is bound to the input field, allowing the user to update the name dynamically.
Techniques for One-Way Data Binding
Angular provides multiple techniques for one-way data binding to suit different scenarios. Let's explore two of the most commonly used techniques:
1) Interpolation Binding: Interpolation binding allows you to bind data values directly into the text of an HTML element using double curly braces {{ }}. It is the simplest form of one-way data binding.
Example: Continuing from the previous example, let's use interpolation to display the user's name in a greeting message.
app.component.html:
<p>Hello, {{ name }}! Welcome to Infital.</p>
2) Property Binding: Property binding is used to set the value of an HTML attribute based on the value of a component's property. This technique is helpful when you want to conditionally modify an element's behavior or appearance.
Example: Suppose we have a button in our component, and we want to disable it when a certain condition is met.
app.component.ts:
export class AppComponent {
isLoggedIn = false;
}
app.component.html:
<button [disabled]="!isLoggedIn">Click me</button>
In this example, the "disabled" attribute of the button is bound to the "isLoggedIn" property using square brackets [ ]. The button will be disabled when "isLoggedIn" is false.
Techniques for Two-Way Data
Binding Similar to one-way data binding, Angular offers different approaches for implementing two-way data binding. Let's explore two popular techniques:
1) ngModel Directive: As we saw in the previous example, the ngModel directive is used to achieve two-way data binding. It allows us to bind an input control with a component property, making data synchronization seamless.
Example: Let's extend the previous example and add a text area where the user can enter additional information.
app.component.ts:
export class AppComponent {
message = "Default message";
}
app.component.html:
<textarea [(ngModel)]="message" placeholder="Enter your message"></textarea>
<h3>Your message: {{ message }}</h3>
In this example, the "message" property is bound to the text area using the ngModel directive, enabling two-way data binding.
2) Event Binding: Event binding is another way to achieve two-way data binding, allowing you to respond to user actions and update the component's data accordingly.
Example: Let's create a button that increments a counter when clicked.
app.component.ts:
export class AppComponent {
counter = 0;
incrementCounter() {
this.counter++;
}
}
app.component.html:
<button (click)="incrementCounter()">Click to Increment</button>
<p>Counter Value: {{ counter }}</p>
In this example, we use the (click) event binding to call the "incrementCounter()" method when the button is clicked. This updates the "counter" property, and the new value is automatically displayed in the view.
Conclusion
Angular data binding plays a pivotal role in creating dynamic and interactive web applications. Understanding the nuances of one-way and two-way data binding is crucial for any Angular developer. In this article, we explored the concepts of both techniques and demonstrated various binding methods with coding examples. Armed with this knowledge, you can now harness the full potential of Angular data binding and build powerful applications that respond seamlessly to user interactions. Happy coding!