ADO.NET vs Entity Framework: Unraveling the Best Data Access Approach
ADO.NET is a robust choice for developers who seek fine-grained control over their data operations, while EF excels in providing an abstraction layer over the database.
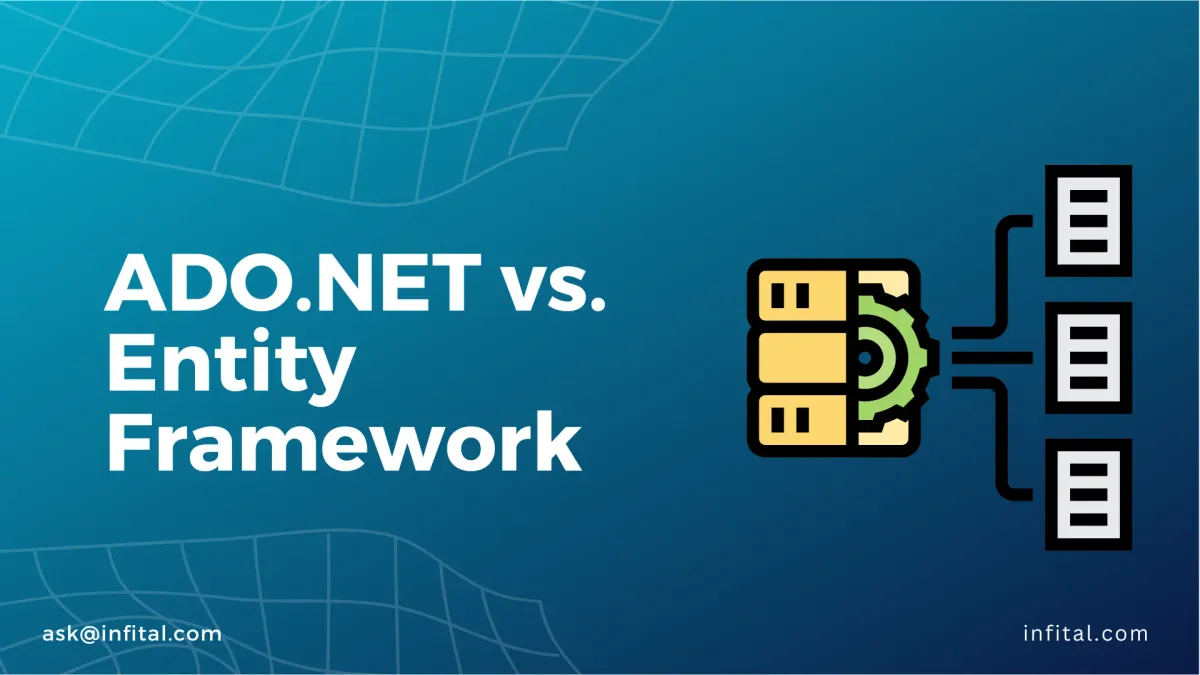
When it comes to developing data-driven applications, developers face a crucial decision: choosing the right data access technology. Two prominent options that often come into the spotlight are ADO.NET and Entity Framework. In this article, we will delve deep into the features, advantages, and use cases of both ADO.NET and Entity Framework to help you make an informed decision for your next project.
Understanding ADO.NET
ADO.NET, developed by Microsoft as part of the .NET Framework, is a powerful data access technology that enables developers to interact with various data sources through their applications. Its primary goal is to provide an object-oriented approach to access databases, ensuring high performance, reliability, and scalability.
Disconnected Architecture: One of the key features of ADO.NET is its disconnected architecture. In this model, a connection to the data source is established only when the application needs to read or update data. This approach significantly reduces resource consumption in client-server or distributed applications, as the connection is opened only when necessary.
Example Code - Connecting to a Database in ADO.NET:
using System;
using System.Data;
using System.Data.SqlClient;
public void ConnectToDatabase()
{
string connectionString = "Data Source=YourServer;Initial Catalog=YourDatabase;Integrated Security=True";
using (SqlConnection connection = new SqlConnection(connectionString))
{
connection.Open();
// Perform database operations here
connection.Close();
}
}
Query Execution and Data Retrieval: With ADO.NET, developers can execute SQL queries and stored procedures to perform read, write, update, and delete operations from the data source. The data is returned in the form of DataReader or DataSet objects, allowing developers to work with the data even after the connection is closed. The DataSet is a container of multiple DataTable objects, and relationships can be established among them, providing a structured representation of the data.
Example Code - Executing a SQL Query with ADO.NET:
using System;
using System.Data;
using System.Data.SqlClient;
public void FetchDataFromDatabase()
{
string connectionString = "Data Source=YourServer;Initial Catalog=YourDatabase;Integrated Security=True";
string sqlQuery = "SELECT * FROM Customers";
using (SqlConnection connection = new SqlConnection(connectionString))
{
SqlCommand command = new SqlCommand(sqlQuery, connection);
connection.Open();
SqlDataReader dataReader = command.ExecuteReader();
while (dataReader.Read())
{
// Process data here
}
dataReader.Close();
connection.Close();
}
}
Introducing Entity Framework
Entity Framework (EF) is another data access technology provided by Microsoft, specifically designed to work with .NET applications. It is an Object-Relational Mapping (ORM) framework, which means it enables developers to interact with databases using object-oriented programming concepts.
Object-Relational Mapping (ORM): Entity Framework bridges the gap between the relational data model of the database and the object-oriented data model of the application. It allows developers to work with entities and relationships directly in their code, abstracting the underlying database details.
Example Code - Defining an Entity Class with Entity Framework:
using System;
using System.ComponentModel.DataAnnotations;
public class Customer
{
[Key]
public int Id { get; set; }
public string Name { get; set; }
public string Email { get; set; }
}
LINQ Support: Entity Framework provides robust support for Language-Integrated Query (LINQ), enabling developers to write database queries using C# or VB.NET syntax. This approach leads to more readable and maintainable code as the queries are written in a language familiar to developers.
Example Code - LINQ Query with Entity Framework:
using System;
using System.Linq;
public void FetchDataWithEF()
{
using (var context = new YourDbContext())
{
var customers = context.Customers.Where(c => c.Age > 18).ToList();
// Process the retrieved data here
}
}
Advantages and Use Cases
Both ADO.NET and Entity Framework have their strengths and use cases.
ADO.NET Advantages:
- Well-established and widely used data access technology.
- Allows fine-grained control over SQL queries and stored procedures.
- Ideal for scenarios where a disconnected architecture is preferred.
- Suitable for applications with complex database operations.
Entity Framework Advantages:
- Simplifies data access with its ORM capabilities.
- LINQ support enhances code readability.
- Speeds up development by reducing boilerplate code.
- Ideal for applications with complex object-oriented data models.
Conclusion
In conclusion, the choice between ADO.NET and Entity Framework depends on your project requirements and development preferences. ADO.NET is a robust choice for developers who seek fine-grained control over their data operations, while Entity Framework excels in providing an abstraction layer over the database and simplifying data access with its ORM features. We hope this article has provided you with a clear understanding of the capabilities and strengths of both technologies, allowing you to make an informed decision for your next data-driven application. Happy coding!