Arrays vs. Lists in C#: Understanding the Key Differences for Efficient Programming
Lists can dynamically grow or shrink as needed and Arrays tend to be more memory efficient than lists. Also the dynamic resizing of lists allows for efficient memory management
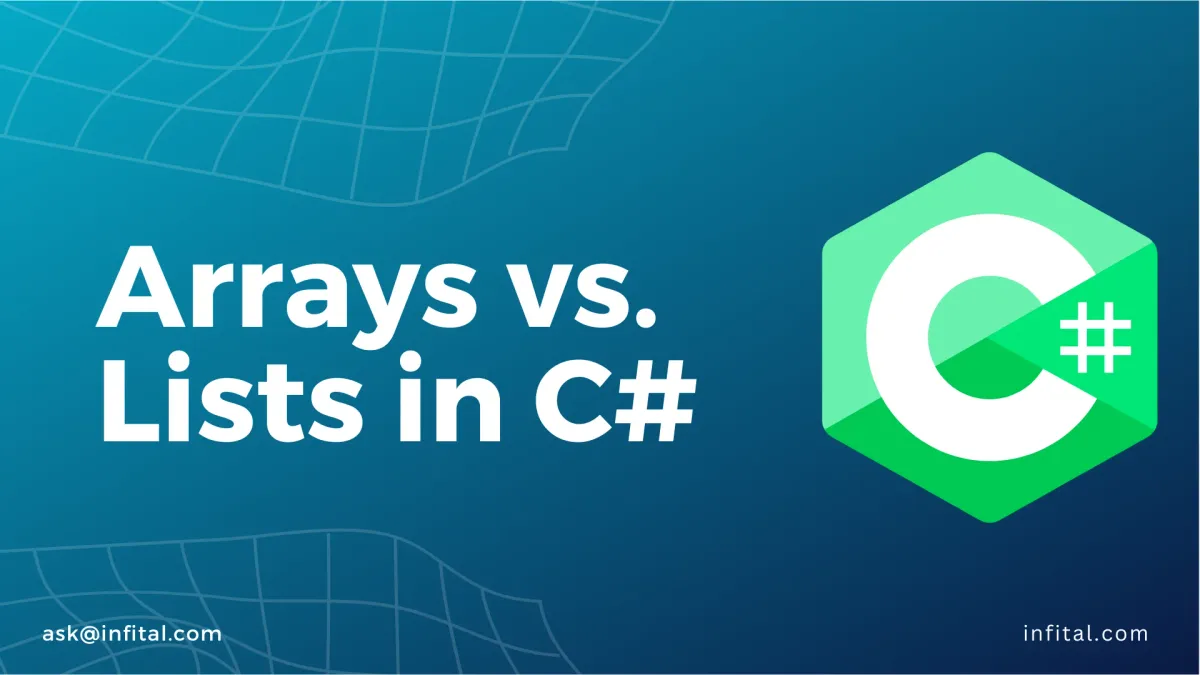
When writing code in C# or any other programming language, you'll often come across the need to store and manipulate collections of data. Two common data structures used for this purpose are arrays and lists. Although they may seem similar at first glance, arrays and lists have fundamental differences that can significantly impact your program's performance and functionality. In this article, we'll delve into the world of arrays and lists, exploring their definitions, purposes, and crucial distinctions to equip you with a deeper understanding of these essential data structures in C#.
Understanding Lists in C#
A list is an ordered collection of elements, and it serves as a versatile data structure in C#. When you want to store a dynamic set of values, such as a list of user names or a collection of book titles, lists come to the rescue. Here's how you can use lists effectively in your C# programs:
Creating and Initializing Lists: To begin using lists, you'll first need to import the System.Collections.Generic
namespace. Then, you can create and initialize a list of integers as follows:
using System.Collections.Generic;
// Creating a list of integers
List<int> numbersList = new List<int> { 1, 2, 3, 4, 5 };
Adding and Removing Elements: Lists provide a straightforward way to add or remove elements. You can append an item to the end of the list using the Add()
method and remove an item using the Remove()
method:
// Adding an element to the list
numbersList.Add(6);
// Removing an element from the list
numbersList.Remove(3);
Accessing Elements: You can access list elements by their index, starting from zero. For instance, to access the third element in the list:
int thirdElement = numbersList[2];
Understanding Arrays in C#
Arrays, like lists, allow you to store collections of elements. However, they have some distinct characteristics that make them suitable for specific scenarios. Let's explore how arrays work and when to use them in your C# code:
Declaring and Initializing Arrays: To create an array, you specify its type and size. Unlike lists, arrays have a fixed length that cannot be changed once defined. Here's how you declare and initialize an array of integers:
// Declaring and initializing an integer array
int[] numbersArray = new int[5] { 1, 2, 3, 4, 5 };
Fixed Size: One critical aspect of arrays is their fixed size. Once you define an array with a specific length, you cannot add or remove elements directly. You would need to create a new array with the desired size and copy elements if you need to resize it.
Indexing and Accessing Elements: Like lists, arrays also use zero-based indexing. To access an element in the array, you use its index:
int secondElement = numbersArray[1];
Key Differences between Lists and Arrays
Now that we've explored both lists and arrays in C#, let's dive into the fundamental differences that set them apart and determine when to use each:
Dynamic vs. Fixed Size: One of the most significant distinctions between lists and arrays is their sizing flexibility. Lists can dynamically grow or shrink as needed, making them suitable for scenarios where the number of elements may change over time. On the other hand, arrays have a fixed size, making them ideal when you know the exact number of elements you'll be working with and want to ensure a specific memory allocation.
Memory Overhead: Arrays tend to be more memory efficient than lists, especially when dealing with a large number of elements. Lists require extra memory to manage their dynamic size and provide additional methods for element manipulation, resulting in a slightly higher memory overhead.
Performance Considerations: For scenarios that involve frequent insertions or deletions of elements, lists generally outperform arrays. The dynamic resizing of lists allows for efficient memory management, reducing the overhead of resizing operations. However, arrays excel in situations that require constant and direct access to elements, as they offer faster element retrieval due to their contiguous memory allocation.
Use Cases and Best Practices
Understanding the differences between lists and arrays enables you to make informed decisions when choosing the appropriate data structure for your C# programs. Consider the following use cases and best practices:
Use Lists When...
- You need a flexible data structure that can grow or shrink dynamically.
- You frequently add or remove elements from the collection.
- You want the convenience of built-in methods for element manipulation.
Use Arrays When...
- You have a fixed number of elements and want to allocate memory efficiently.
- You need to access elements with high performance and predictability.
- You are dealing with a large dataset, and memory usage is a critical concern.
Conclusion
Arrays and lists are essential data structures in C#, each with its unique characteristics and use cases. By understanding their differences, you can make informed decisions and write more efficient and optimized code. Whether you need the dynamic capabilities of lists or the predictable performance of arrays, C# offers you the tools to handle collections effectively and enhance your programming skills. Happy coding!