Mastering Dictionary in C#: A Comprehensive Guide for Beginners
Much like a non-generic hashtable, a Dictionary is a generic collection. What sets it apart is its generic nature, lending it the ability to work with various data types.
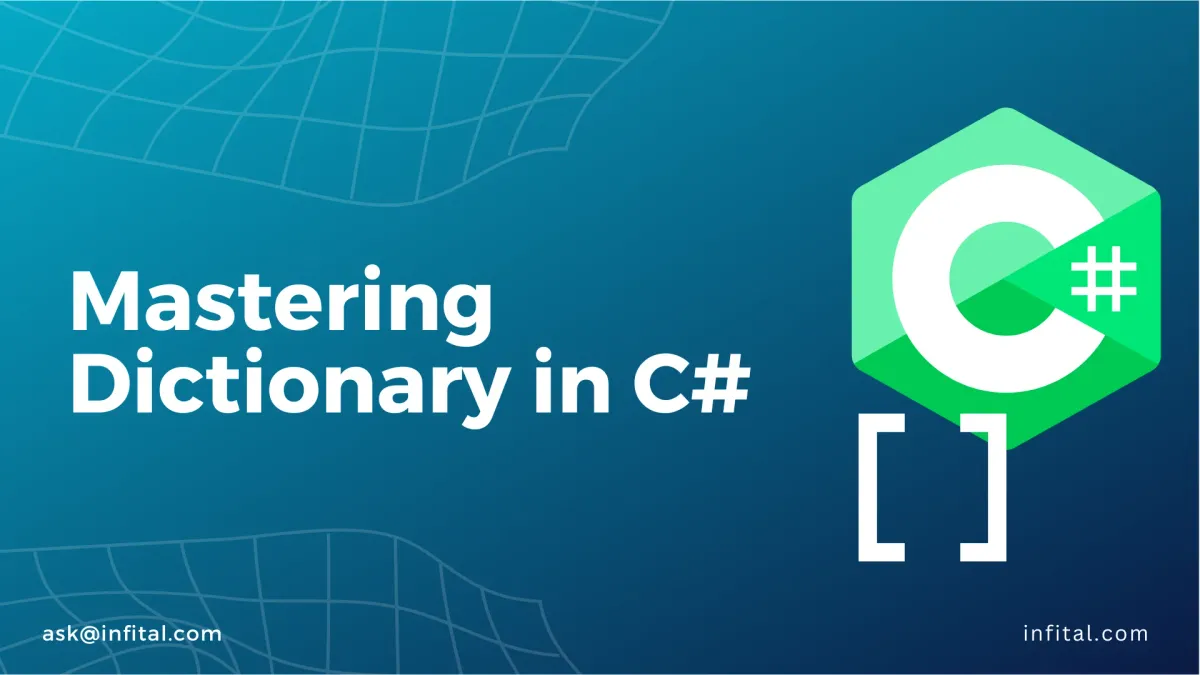
In the realm of C# programming, mastering data storage and retrieval is paramount. Enter the Dictionary class, a dynamic and versatile collection that empowers developers to efficiently manage key/value pairs. Whether you're an aspiring programmer or a curious enthusiast, this in-depth guide will unravel the intricacies of Dictionaries in C#, offering you a firm grasp of their creation, manipulation, and utilization.
The Foundation of Dictionaries
Before we delve into the depths, let's establish a solid foundation by comprehending the fundamental aspects of Dictionaries in C#. Much like a non-generic hashtable, a Dictionary is a generic collection. What sets it apart is its generic nature, lending it the ability to work with various data types. This powerful tool resides under the System.Collections.Generic namespace, boasting the capacity to dynamically adjust its size according to the requirements, ensuring optimal memory utilization.
Crafting Your Dictionary
Creating a Dictionary in C# is a breeze, thanks to the Dictionary<TKey, TValue>() constructor. Here's a step-by-step breakdown:
using System.Collections.Generic;
// Creating a Dictionary
Dictionary<string, int> studentScores = new Dictionary<string, int>();
In this example, we initiate an empty Dictionary ready to house string keys and integer values.
Breathing Life into Your Dictionary
Our Dictionary is now ready, and it's time to infuse it with data. The Add()
method is our conduit to achieve this:
studentScores.Add("Alice", 95);
studentScores.Add("Bob", 82);
Retrieving values is a breeze as well:
int aliceScore = studentScores["Alice"];
But remember, the uniqueness of keys is of utmost importance. Attempting to insert a duplicate key will result in an exception, underscoring the significance of maintaining distinct keys.
Navigating the Dictionary Terrain
Embarking on a journey through your Dictionary is an illuminating experience. One effective method involves employing a foreach
loop:
foreach (KeyValuePair<string, int> kvp in studentScores)
{
Console.WriteLine($"Name: {kvp.Key}, Score: {kvp.Value}");
}
For those inclined towards a more traditional approach, a for
loop can also be employed, with the Count
property ensuring accurate traversal:
for (int i = 0; i < studentScores.Count; i++)
{
Console.WriteLine($"Name: {studentScores.Keys.ElementAt(i)}, Score: {studentScores.Values.ElementAt(i)}");
}
Unburdening the Dictionary
Bid farewell to unwanted entries with ease. The Clear()
method performs the honorable duty of wiping the Dictionary clean:
studentScores.Clear();
Should a specific entry be the object of your attention, the Remove()
method proves invaluable:
studentScores.Remove("Alice");
In the pursuit of efficient code, these methods play a pivotal role.
Unveiling Existence
The ability to verify the presence of elements within your Dictionary is indispensable. Armed with the ContainsKey()
and ContainsValue()
methods, you can ascertain their existence with elegance:
if (studentScores.ContainsKey("Bob"))
{
// Bob's score resides in the Dictionary
}
if (studentScores.ContainsValue(82))
{
// A score of 82 finds its abode in the Dictionary
}
Safeguarding Against KeyNotFoundException
Errant keys can lead to exceptions, disrupting the harmony of your code. The TryGetValue()
method acts as a shield, offering protection against the dreaded KeyNotFoundException
:
if (studentScores.TryGetValue("Charlie", out int charlieScore))
{
Console.WriteLine($"Charlie's Score: {charlieScore}");
}
else
{
Console.WriteLine("Charlie's score is shrouded in mystery.");
}
Culmination and Future Endeavors
Congratulations, you've traversed the labyrinth of Dictionaries in C#. Armed with a profound understanding of creation, manipulation, and exploration, you're primed to elevate your C# journey. Dictionaries are not merely data structures; they're your accomplices in crafting elegant and efficient code.
Conclusion
In this comprehensive journey, we've unfurled the tapestry of Dictionaries in C#. From inception to removal, traversal to verification, you've acquired a holistic comprehension of this versatile tool. As you embark on future coding exploits, Dictionaries will remain a steadfast ally, propelling your projects to new heights. Embrace this newfound knowledge, and let the world of Dictionaries become an integral part of your coding odyssey. Happy coding!